diff --git a/src/plugins/fullSearchContext/README.md b/src/plugins/fullSearchContext/README.md
new file mode 100644
index 000000000..4bc5feb5a
--- /dev/null
+++ b/src/plugins/fullSearchContext/README.md
@@ -0,0 +1,5 @@
+# FullSearchContext
+
+Makes the message context menu in message search results have all options you'd expect.
+
+
diff --git a/src/plugins/fullSearchContext/index.tsx b/src/plugins/fullSearchContext/index.tsx
new file mode 100644
index 000000000..158612c6a
--- /dev/null
+++ b/src/plugins/fullSearchContext/index.tsx
@@ -0,0 +1,82 @@
+/*
+ * Vencord, a modification for Discord's desktop app
+ * Copyright (c) 2023 Vendicated and contributors
+ *
+ * This program is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+ * GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+*/
+
+import { migratePluginSettings } from "@api/Settings";
+import { Devs } from "@utils/constants";
+import definePlugin from "@utils/types";
+import { findByPropsLazy } from "@webpack";
+import { ChannelStore, ContextMenuApi, i18n, UserStore } from "@webpack/common";
+import { Message } from "discord-types/general";
+import type { MouseEvent } from "react";
+
+const { useMessageMenu } = findByPropsLazy("useMessageMenu");
+
+function MessageMenu({ message, channel, onHeightUpdate }) {
+ const canReport = message.author &&
+ !(message.author.id === UserStore.getCurrentUser().id || message.author.system);
+
+ return useMessageMenu({
+ navId: "message-actions",
+ ariaLabel: i18n.Messages.MESSAGE_UTILITIES_A11Y_LABEL,
+
+ message,
+ channel,
+ canReport,
+ onHeightUpdate,
+ onClose: () => ContextMenuApi.closeContextMenu(),
+
+ textSelection: "",
+ favoriteableType: null,
+ favoriteableId: null,
+ favoriteableName: null,
+ itemHref: void 0,
+ itemSrc: void 0,
+ itemSafeSrc: void 0,
+ itemTextContent: void 0,
+ });
+}
+
+migratePluginSettings("FullSearchContext", "SearchReply");
+export default definePlugin({
+ name: "FullSearchContext",
+ description: "Makes the message context menu in message search results have all options you'd expect",
+ authors: [Devs.Ven, Devs.Aria],
+
+ patches: [{
+ find: "onClick:this.handleMessageClick,",
+ replacement: {
+ match: /this(?=\.handleContextMenu\(\i,\i\))/,
+ replace: "$self"
+ }
+ }],
+
+ handleContextMenu(event: MouseEvent, message: Message) {
+ const channel = ChannelStore.getChannel(message.channel_id);
+ if (!channel) return;
+
+ event.stopPropagation();
+
+ ContextMenuApi.openContextMenu(event, contextMenuProps =>
+
+ );
+ }
+});
diff --git a/src/plugins/searchReply/README.md b/src/plugins/searchReply/README.md
deleted file mode 100644
index b06798f74..000000000
--- a/src/plugins/searchReply/README.md
+++ /dev/null
@@ -1,6 +0,0 @@
-# SearchReply
-
-Adds a reply button to search results.
-
-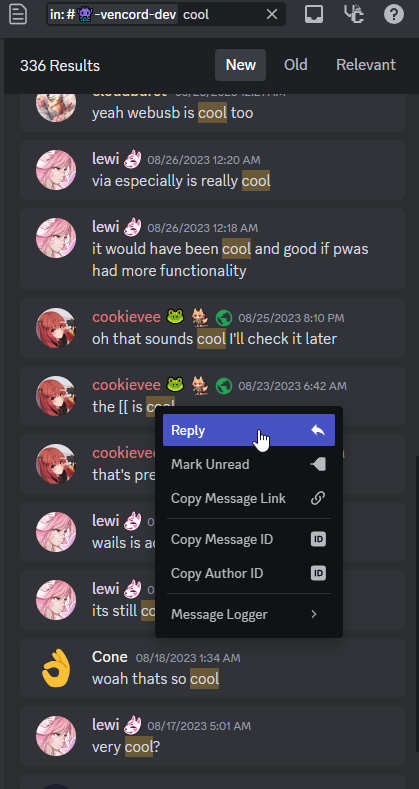
-
diff --git a/src/plugins/searchReply/index.tsx b/src/plugins/searchReply/index.tsx
deleted file mode 100644
index 298e74218..000000000
--- a/src/plugins/searchReply/index.tsx
+++ /dev/null
@@ -1,75 +0,0 @@
-/*
- * Vencord, a modification for Discord's desktop app
- * Copyright (c) 2023 Vendicated and contributors
- *
- * This program is free software: you can redistribute it and/or modify
- * it under the terms of the GNU General Public License as published by
- * the Free Software Foundation, either version 3 of the License, or
- * (at your option) any later version.
- *
- * This program is distributed in the hope that it will be useful,
- * but WITHOUT ANY WARRANTY; without even the implied warranty of
- * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
- * GNU General Public License for more details.
- *
- * You should have received a copy of the GNU General Public License
- * along with this program. If not, see .
-*/
-
-import { findGroupChildrenByChildId, NavContextMenuPatchCallback } from "@api/ContextMenu";
-import { ReplyIcon } from "@components/Icons";
-import { Devs } from "@utils/constants";
-import definePlugin from "@utils/types";
-import { findByCodeLazy } from "@webpack";
-import { ChannelStore, i18n, Menu, PermissionsBits, PermissionStore, SelectedChannelStore } from "@webpack/common";
-import { Message } from "discord-types/general";
-
-
-const replyToMessage = findByCodeLazy(".TEXTAREA_FOCUS)", "showMentionToggle:");
-
-const messageContextMenuPatch: NavContextMenuPatchCallback = (children, { message }: { message: Message; }) => {
- // make sure the message is in the selected channel
- if (SelectedChannelStore.getChannelId() !== message.channel_id) return;
- const channel = ChannelStore.getChannel(message?.channel_id);
- if (!channel) return;
- if (channel.guild_id && !PermissionStore.can(PermissionsBits.SEND_MESSAGES, channel)) return;
-
- // dms and group chats
- const dmGroup = findGroupChildrenByChildId("pin", children);
- if (dmGroup && !dmGroup.some(child => child?.props?.id === "reply")) {
- const pinIndex = dmGroup.findIndex(c => c?.props.id === "pin");
- dmGroup.splice(pinIndex + 1, 0, (
-
replyToMessage(channel, message, e)}
- />
- ));
- return;
- }
-
- // servers
- const serverGroup = findGroupChildrenByChildId("mark-unread", children);
- if (serverGroup && !serverGroup.some(child => child?.props?.id === "reply")) {
- serverGroup.unshift((
- replyToMessage(channel, message, e)}
- />
- ));
- return;
- }
-};
-
-
-export default definePlugin({
- name: "SearchReply",
- description: "Adds a reply button to search results",
- authors: [Devs.Aria],
- contextMenus: {
- "message": messageContextMenuPatch
- }
-});